Vue Js Generate Hash Password:Vue.js is a front-end JavaScript framework that allows developers to build web applications. One of the common tasks when building web applications is to generate a hash password, which can be done using a built-in method in JavaScript called crypto.subtle.digest()
. This method takes the password string as input, then generates a hash of the password using a cryptographic hash function, such as SHA-256. The resulting hash can be stored in a database instead of the actual password, which provides an additional layer of security in case of a data breach. Vue.js can easily integrate this method into its components and provide a secure way to store and manage user passwords.
How can Vue Js be used to generate a hash password?
The code you provided is a Vue.js method for generating a hash password using the SHA-256 algorithm. Here is a brief explanation of how it works:
- The method takes the value of the password from the
this.password
property. - The
TextEncoder
constructor is used to create an encoder object for converting the password to aUint8Array
. - The
encoder.encode()
method is called with the password as its parameter to convert the password to aUint8Array
. - The
crypto.subtle.digest()
method is used to generate a hash of the password using the SHA-256 algorithm. - The
await
keyword is used to wait for the promise returned by thedigest()
method to be resolved. - The resulting hash is returned as an ArrayBuffer object.
- The
Array.from()
method is used to convert the ArrayBuffer to an array of bytes. - The
map()
method is used to convert each byte to its hexadecimal representation. - The
padStart()
method is used to ensure that each hexadecimal representation has two characters. - The resulting array of hexadecimal representations is joined into a single string using the
join()
method. - The resulting hash password string is assigned to the
this.hashPassword
property.
Overall, this method takes a plaintext password and generates a hashed password using the SHA-256 algorithm, which is commonly used for secure password storage.
Vue Js Generate Hash Password Example
<div id="app">
<form @submit.prevent="generateHashPass">
<label for="password">Password:</label>
<input type="password" id="password" v-model="password" />
<button type="submit">Generate Hash Password</button>
<small v-if="hashPassword">Hash Password: {{hashPassword}}</small>
</form>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
password: "123456789",
hashPassword: ''
};
},
methods: {
async generateHashPass() {
const password = this.password;
const encoder = new TextEncoder();
const data = encoder.encode(password);
const hash = await crypto.subtle.digest("SHA-256", data);
const hashArray = Array.from(new Uint8Array(hash));
const hashHex = hashArray.map((b) => b.toString(16).padStart(2, "0")).join("");
this.hashPassword = hashHex
},
},
})
</script>
Output of Vue Js Generate Hash Password
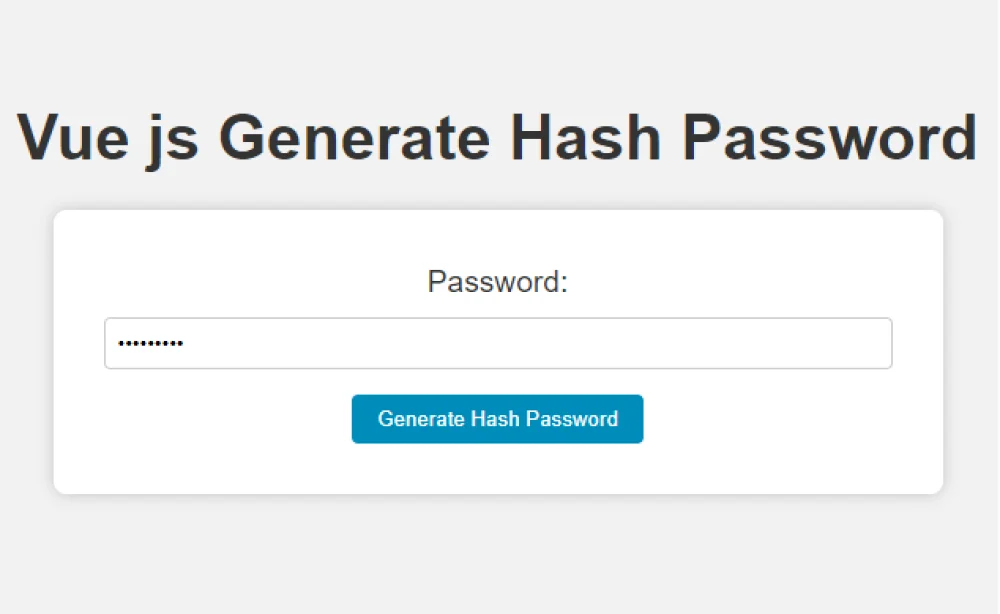